| |
illustrates the use of a Stack |
|
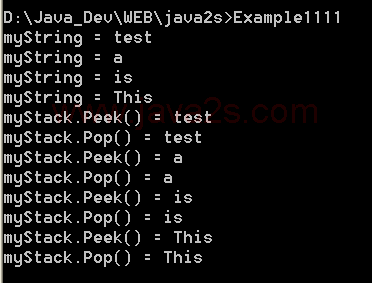 |
/*
Mastering Visual C# .NET
by Jason Price, Mike Gunderloy
Publisher: Sybex;
ISBN: 0782129110
*/
/*
Example11_11.cs illustrates the use of a Stack
*/
using System;
using System.Collections;
public class Example11_11
{
public static void Main()
{
// create a Stack object
Stack myStack = new Stack();
// add four elements to myStack using the Push() method
myStack.Push("This");
myStack.Push("is");
myStack.Push("a");
myStack.Push("test");
// display the elements in myStack
foreach (string myString in myStack)
{
Console.WriteLine("myString = " + myString);
}
// get the number of elements in myStack using the
// Count property
int numElements = myStack.Count;
for (int count = 0; count < numElements; count++)
{
// examine an element in myStack using Peek()
Console.WriteLine("myStack.Peek() = " +
myStack.Peek());
// remove an element from myStack using Pop()
Console.WriteLine("myStack.Pop() = " +
myStack.Pop());
}
}
}
|
|
|
Related examples in the same category |
|