| |
Encode or decode a message |
|
/*
C#: The Complete Reference
by Herbert Schildt
Publisher: Osborne/McGraw-Hill (March 8, 2002)
ISBN: 0072134852
*/
// Encode or decode a message.
using System;
public class Cipher {
public static int Main(string[] args) {
// see if arguments a present
if(args.Length < 2) {
Console.WriteLine("Usage: encode/decode word1 [word2...wordN]");
return 1; // return failure code
}
// if args present, first arg must be encode or decode
if(args[0] != "encode" & args[0] != "decode") {
Console.WriteLine("First arg must be encode or decode.");
return 1; // return failure code
}
// encode or decode message
for(int n=1; n < args.Length; n++) {
for(int i=0; i < args[n].Length; i++) {
if(args[0]=="encode")
Console.Write((char) (args[n][i] + 1) );
else
Console.Write((char) (args[n][i] - 1) );
}
Console.Write(" ");
}
Console.WriteLine();
return 0;
}
}
|
|
|
Related examples in the same category |
1. | Get char type: control, digit, letter, number, punctuation, surrogate, symbol and white space | | 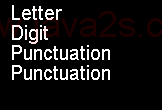 | 2. | Determining If A Character Is Within A Specified Range | | 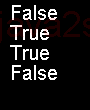 | 3. | Is a char in a range: Case Insensitive | | 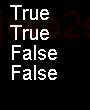 | 4. | Is a char in a range Exclusively | |  | 5. | Using Char | | | 6. | Escape Characters | | | 7. | A stack class for characters | | 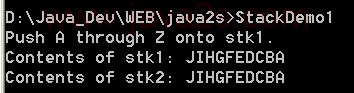 | 8. | Demonstrate several Char methods | | 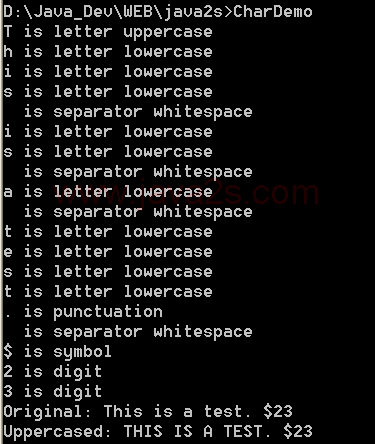 | 9. | Demonstrate the ICharQ interface: A character queue interface | | 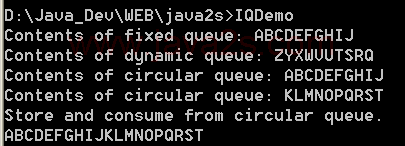 | 10. | A set class for characters | | 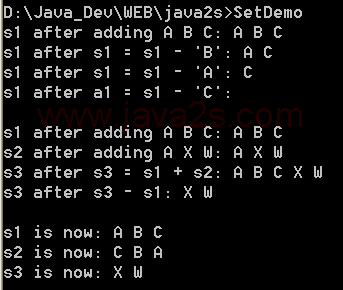 | 11. | A queue class for characters | | 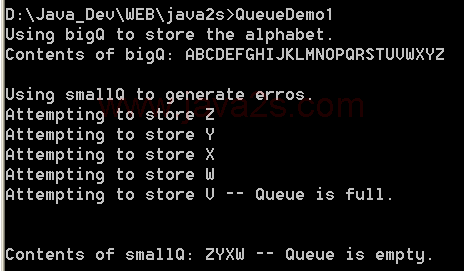 | 12. | IsDigit, IsLetter, IsWhiteSpace, IsLetterOrDigit, IsPunctuation | | | 13. | Char: Get Unicode Category | | | 14. | Char.IsLowSurrogate(), IsHighSurrogate(), IsSurrogatePair() method | | | 15. | demonstrates IsSymbol. | | | 16. | Buffer for characters | | | 17. | Test an input character if it is contained in a character list. | | | 18. | Is vowel char | | |
|