| |
Implement the Pythagorean Theorem |
|
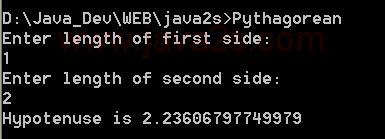 |
/*
C#: The Complete Reference
by Herbert Schildt
Publisher: Osborne/McGraw-Hill (March 8, 2002)
ISBN: 0072134852
*/
// Implement the Pythagorean Theorem.
using System;
public class Pythagorean {
public static void Main() {
double s1;
double s2;
double hypot;
string str;
Console.WriteLine("Enter length of first side: ");
str = Console.ReadLine();
s1 = Double.Parse(str);
Console.WriteLine("Enter length of second side: ");
str = Console.ReadLine();
s2 = Double.Parse(str);
hypot = Math.Sqrt(s1*s1 + s2*s2);
Console.WriteLine("Hypotenuse is " + hypot);
}
}
|
|
|
Related examples in the same category |
1. | Compute the area of a circle | |  | 2. | the differences
between int and double | |  | 3. | Talking to Mars: double value calculation | |  | 4. | converts Fahrenheit to Celsius | |  | 5. | Epsilon, PositiveInfinity, NegativeInfinity, MaxValue, MinValue | | | 6. | double number format: 0:C, 0:D9, 0:E, 0:F3, 0:N, 0:X, 0:x | | | 7. | Format double value | | | 8. | Get Decimal Places | | | 9. | Automatic conversion from double to string | | | 10. | An int, a short, a float, and a double are added together giving a double result. | | | 11. | Test to see if a double is a finite number (is not NaN or Infinity). | | |
|