| |
Thread DeadLock: Philosopher |
|
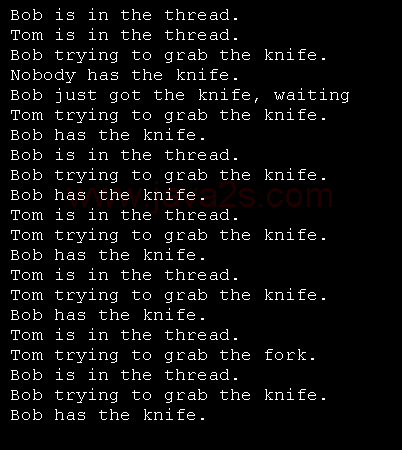 |
Imports System
Imports System.Threading
Imports System.Text
Imports System.Windows.Forms
Public Class MainClass
Public Shared Sub Main()
Dim Tom As New Philosopher("Tom")
Dim Bob As New Philosopher("Bob")
Dim aThreadStart As New ThreadStart(AddressOf Tom.Eat)
Dim aThread As New Thread(aThreadStart)
aThread.Name = "Tom"
Dim bThreadStart As New ThreadStart(AddressOf Bob.Eat)
Dim bThread As New Thread(bThreadStart)
bThread.Name = "Bob"
aThread.Start()
bThread.Start()
End Sub
End Class
Public Class Fork
Private Shared mForkAvailable As Boolean = True
Private Shared OwnerName As String = "Nobody"
Public Sub GrabFork(ByVal a As Philosopher)
Console.WriteLine(Thread.CurrentThread.Name & " trying to grab the fork.")
Console.WriteLine(Me.OwnerName & " has the fork.")
Monitor.Enter(Me) 'SyncLock (aFork) '
If mForkAvailable Then
a.HasFork = True
OwnerName = a.MyName
mForkAvailable = False
Console.WriteLine(a.MyName & " just got the fork, waiting")
Thread.Sleep(100)
End If
Monitor.Exit(Me) 'End SyncLock
End Sub
End Class
Public Class Knife
Private Shared mKnifeAvailable As Boolean = True
Private Shared OwnerName As String = "Nobody"
Public Sub GrabKnife(ByVal a As Philosopher)
Console.WriteLine(Thread.CurrentThread.Name & " trying to grab the knife.")
Console.WriteLine(Me.OwnerName & " has the knife.")
Monitor.Enter(Me) 'SyncLock (aKnife) '
If mKnifeAvailable Then
mKnifeAvailable = False
a.HasKnife = True
OwnerName = a.MyName
Console.WriteLine(a.MyName & " just got the knife, waiting")
Thread.Sleep(100)
End If
Monitor.Exit(Me)
End Sub
End Class
Public Class Philosopher
Public MyName As String
Private Shared mFork As Fork
Private Shared mKnife As Knife
Public HasKnife As Boolean
Public HasFork As Boolean
Shared Sub New()
mFork = New Fork()
mKnife = New Knife()
End Sub
Public Sub New(ByVal theName As String)
MyName = theName
End Sub
Public Sub Eat()
Do Until Me.HasKnife And Me.HasFork
Console.WriteLine(Thread.CurrentThread.Name & " is in the thread.")
If Rnd() < 0.5 Then
mFork.GrabFork(Me)
Else
mKnife.GrabKnife(Me)
End If
Loop
MessageBox.Show(Me.MyName & " can eat!")
mKnife = New Knife()
mFork = New Fork()
End Sub
End Class
|
|
|
Related examples in the same category |
|