| |
Using functions isspace, iscntrl, ispunct, isprint, isgraph |
|
#include <iostream>
#include <ctype.h>
using namespace std;
int main(){
cout << "According to isspace:\nNewline "
<< ( isspace( '\n' ) ? "is a" : "is not a" )
<< " whitespace character\nHorizontal tab "
<< ( isspace( '\t' ) ? "is a" : "is not a" )
<< " whitespace character\n"
<< ( isspace( '%' ) ? "% is a" : "% is not a" )
<< " whitespace character\n";
cout << "\nAccording to iscntrl:\nNewline "
<< ( iscntrl( '\n' ) ? "is a" : "is not a" )
<< " control character\n"
<< ( iscntrl( '$' ) ? "$ is a" : "$ is not a" )
<< " control character\n";
cout << "\nAccording to ispunct:\n"
<< ( ispunct( ';' ) ? "; is a" : "; is not a" )
<< " punctuation character\n"
<< ( ispunct( 'Y' ) ? "Y is a" : "Y is not a" )
<< " punctuation character\n"
<< ( ispunct('#') ? "# is a" : "# is not a" )
<< " punctuation character\n";
cout << "\nAccording to isprint:\n"
<< ( isprint( '$' ) ? "$ is a" : "$ is not a" )
<< " printing character\nAlert "
<< ( isprint( '\a' ) ? "is a" : "is not a" )
<< " printing character\n";
cout << "\nAccording to isgraph:\n"
<< ( isgraph( 'Q' ) ? "Q is a" : "Q is not a" )
<< " printing character other than a space\nSpace "
<< ( isgraph(' ') ? "is a" : "is not a" )
<< " printing character other than a space" << endl;
return 0;
}
|
|
|
Related examples in the same category |
1. | Wrap char pointer to a String class | |  | 2. | Read string and output its length | | 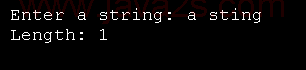 | 3. | Declare a stack class for characters. | | 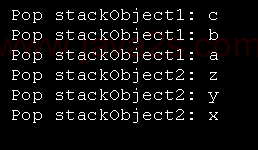 | 4. | Overload string reversal function. | | 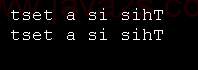 | 5. | Enters a character and outputs its octal, decimal, and hexadecimal code. | | | 6. | cin and cout work with char array | | 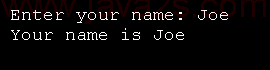 | 7. | Declares str just before it is needed | | 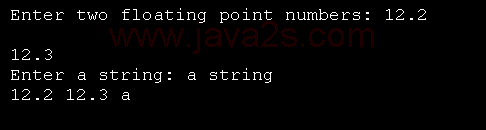 | 8. | Using C strings: cin,=.sync, getline | | 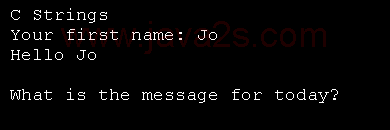 | 9. | Convert a number to a char then convert to upper case and lower case | | | 10. | Using functions islower, isupper, tolower, toupper | | | 11. | Using functions isdigit, isalpha, isalnum, and isxdigit | | |
|