| |
Wrap char pointer to a String class |
|
 |
#include <iostream>
#include <cstring>
#include <cstdlib>
using namespace std;
class StringClass {
char *p;
int len;
public:
StringClass();
StringClass(char *s, int l);
char *getstring() {
return p;
}
int getlength() {
return len;
}
};
StringClass::StringClass()
{
p = new char [255];
if(!p) {
cout << "Allocation error\n";
exit(1);
}
*p = '\0'; // null string
len = 255;
}
StringClass::StringClass(char *s, int l)
{
if(strlen(s) >= l) {
cout << "Allocating too little memory!\n";
exit(1);
}
p = new char [l];
if(!p) {
cout << "Allocation error\n";
exit(1);
}
strcpy(p, s);
len = l;
}
int main()
{
StringClass stringObject1;
StringClass stringObject2("www.java2java.com", 100);
cout << "stringObject1: " << stringObject1.getstring() << " - Length: ";
cout << stringObject1.getlength() << '\n';
cout << "stringObject2: " << stringObject2.getstring() << " - Length: ";
cout << stringObject2.getlength() << '\n';
return 0;
}
|
|
|
Related examples in the same category |
1. | Read string and output its length | | 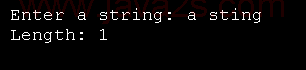 | 2. | Declare a stack class for characters. | | 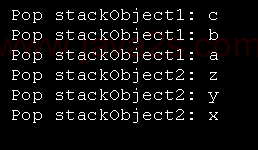 | 3. | Overload string reversal function. | | 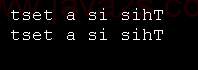 | 4. | Enters a character and outputs its octal, decimal, and hexadecimal code. | | | 5. | cin and cout work with char array | | 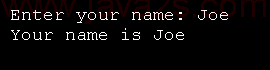 | 6. | Declares str just before it is needed | | 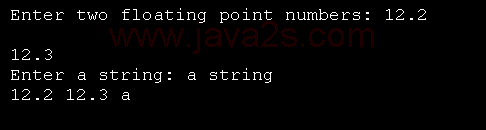 | 7. | Using C strings: cin,=.sync, getline | | 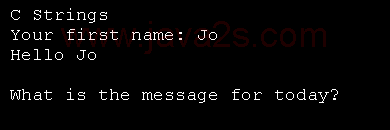 | 8. | Convert a number to a char then convert to upper case and lower case | | | 9. | Using functions islower, isupper, tolower, toupper | | | 10. | Using functions isdigit, isalpha, isalnum, and isxdigit | | | 11. | Using functions isspace, iscntrl, ispunct, isprint, isgraph | | |
|